Web Components Nested Slots
schedule.vue
- Web Components Nested Slots Machines
- Web Components Nested Slots C++
- Web Components Nested Slots Software
- Web Components Nested Slots Folders
<template> |
<divclass='container'style='margin-top: 5rem;'> |
<divclass='row'> |
<divclass='col-md-6 offset-md-3'> |
<ToDoList :items='items'> |
<templatev-slot:todo-item-after='{ item }'> |
<iv-if='isFinished(item)'class='fas fa-check' /> |
</template> |
</ToDoList> |
</div> |
</div> |
</div> |
</template> |
<script> |
importToDoListfrom'~/components/ToDoList' |
import_from'lodash' |
exportdefault { |
components: { ToDoList }, |
data() { |
return { |
items: [ |
{ id:1, title:'todo item 1' }, |
{ id:2, title:'todo item 2' }, |
{ id:3, title:'todo item 3' }, |
{ id:4, title:'todo item 4' } |
], |
finished: [ 1, 2 ] |
} |
}, |
methods: { |
isFinished(item) { |
return_.includes(this.finished, item.id) |
} |
} |
} |
</script> |
- With Shadow DOM, we can create Web Components that are a composition between the component’s internals and elements provided at author-time by the user of the custom element.
- Web components are a set of web platform APIs that allow you to create new custom, reusable and encapsulated HTML tags for use in web pages and web apps. Custom components and widgets are based on web component standards, work on modern browsers and they can be used with any HTML-compatible JavaScript library or framework.
- Now, in this article, we will learn about Angular 2 components and we will also see how to create a nested component and how parent and child components communicate with each other. Angular 2 Components. We all know that Angular 2 is a component based framework because everything is a component in Angular 2.
- In this tutorial, we will implement this dropdown component step by step from scratch with Web Components. Afterward, you can continue using it across your application, make it an open source web component to install it somewhere else, or use a framework like React to build upon a solid foundation of Web Components for your React application.
Web Components Nested Slots Machines
Nested scoped slots in Vue templates. GitHub Gist: instantly share code, notes, and snippets.

TodoItem.vue
<template> |
<li> |
<spanclass='text-muted mr-1'>{{ item.id }}.</span> {{ item.title }} |
<slotname='after' :item='item' /> |
</li> |
</template> |
<script> |
exportdefault { |
props: [ 'item' ] |
} |
</script> |
TodoList.vue
<template> |
<ul> |
<ToDoItemv-for='item in items' :key='item.id' :item='item'> |
<templatev-slot:after='{ item }'> |
<slotname='todo-item-after' :item='item' /> |
</template> |
</ToDoItem> |
</ul> |
</template> |
<script> |
importToDoItemfrom'./ToDoItem' |
exportdefault { |
components: { ToDoItem }, |
props: [ 'items' ] |
} |
</script> |
Sign up for freeto join this conversation on GitHub. Already have an account? Sign in to comment
App.vue
<template> |
<manage-listsv-model='items'> |
<templatescope='{ item: user }'> |
{{ user.firstName }} {{ user.lastName }} |
</template> |
</manage-lists> |
</template> |
<script> |
exportdefault { |
components: { |
ManageLists |
}, |
data() { |
return { |
items: [ |
{ firstName:'John', lastName:'Doe' }, |
{ firstName:'Renae', lastName:'McGillicuddy' } |
] |
}; |
} |
} |
</script> |
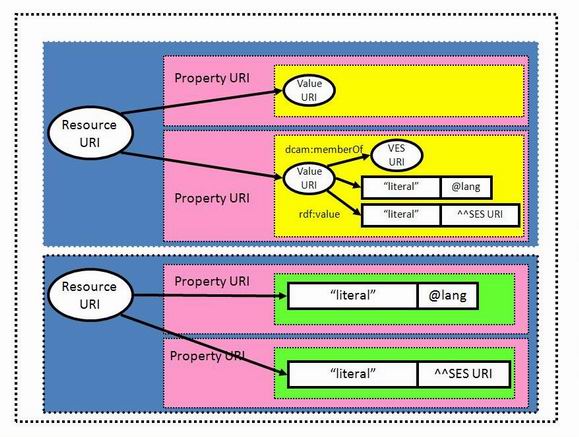

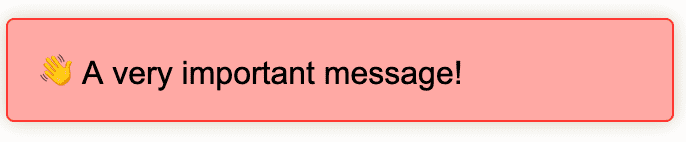
ManageLists.vue
Web Components Nested Slots C++
<template> |
<div> |
<token-list :value='items' @input='forwardInput'> |
<templatescope='props'> |
<slotv-bind='props'></slot> |
</template> |
</token-list> |
<formclass='form' @submit.prevent='handleAdd'> |
<inputtype='text'placeholder='New Item'v-model='newItem'ref='newItem' /> |
<buttontype='submit'>Add Item</button> |
</form> |
</div> |
</template> |
<script> |
importTokenListfrom'./TokenList'; |
exportdefault { |
props: { |
value: { |
type:Array, |
default() { |
return []; |
} |
} |
}, |
components: { |
TokenList |
}, |
methods: { |
handleAdd() { |
this.$emit( 'input', this.value.concat( this.newItem ) ); |
this.newItem=''; |
this.$refs.newItem.focus(); |
}, |
forwardInput( payload ) { |
this.$emit( 'input', payload ); |
} |
} |
} |
</script> |
Web Components Nested Slots Software
TokenList.vue
Web Components Nested Slots Folders
<template> |
<divclass='token-list clearfix'> |
<divv-for='( item, index ) in value'class='token-item'> |
<slot :item='item' :index='index'> |
<span>{{ item }}</span> |
<buttontype='button' @click='remove( index )'>×</button> |
</slot> |
</div> |
</div> |
</template> |
<script> |
exportdefault { |
props: { |
value: { |
required:true |
} |
} |
methods: { |
remove( index ) { |
this.$emit( 'input', [ |
...this.value.slice( 0, index ), |
...this.value.slice( index +1 ) |
] ); |
} |
} |
} |
</script> |
Sign up for freeto join this conversation on GitHub. Already have an account? Sign in to comment